Written guide
Table of contents
Prerequisites
The Node.js JavaScript runtime in required to develop, build and run React applications. You can check the Prerequisites section for the official site link. Unless you need the latest features of Node, it is recommended to use the LTS (Long-Term Support) version as that is the one that will be supported for the longest time.
You will also need a fundamental understanding of HTML, CSS and JavaScript. As you will see throughout your React journey, many of the basic principles of these 3 technologies will be utilized when developing React applications.
Setting up and starting your application
Let's get to configuring! First, set the values for your application in the Configurer Tool above. If you are new to React I recommend starting without TypeScript.
Next, open a terminal (I recommend using bash) and change into a directory of your choosing. You can use the copy button next to each command in the Configurer Tool and simply paste them one after another inside your terminal.
With these commands run you will have set up a brand new React project, and started it's development server (which was the last command). You can view your brand new application at http://localhost:3000, and it should look something like the image below.
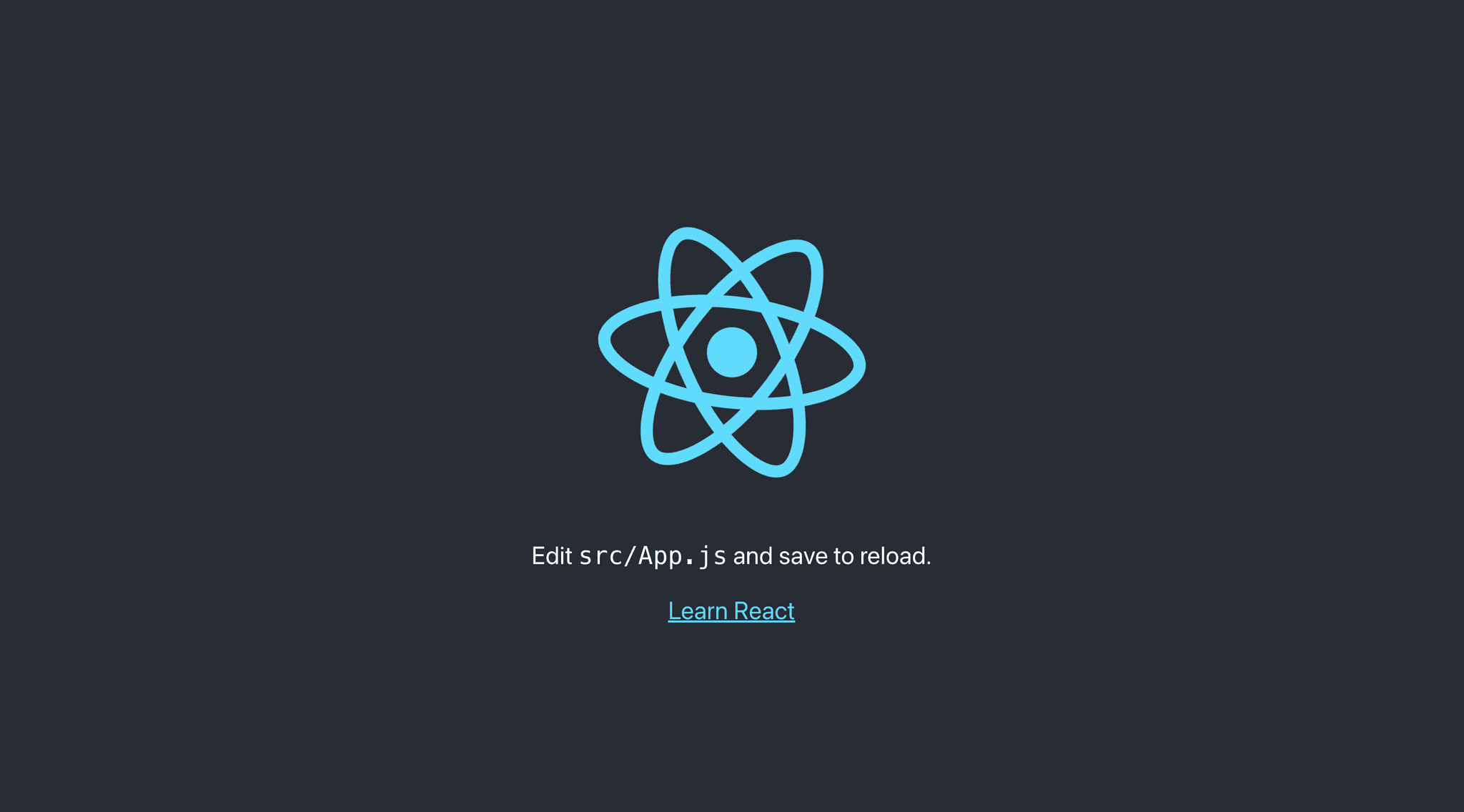
The dev server will start on port 3000 by default. However, it may be the case that you already have something running there. No worries! When starting the application, you will get a message asking you if you would like to start your application on the next available port. Simply hit Y
and the tool will use the next available port.

The dev server
I'd like to briefly touch on this dev server I keep mentioning. Think of it as a tool designed to make the development process easier. With simple HTML pages viewing your latest changes is quite easy: you make changes and reload the browser tab. React is a bit more complicated than and the process behind bundling all your code into an application is more complex. The dev server makes it so we don't have to worry about this bundling process at all. By starting the dev server we get access to immediate feedback of what we have built because it will bundle the project when any of the source files change and reload your browser tab with the newest version of your app. Try it out! While the dev server is running, open the src/App.js
file and change something in it. As soon as you save the file you will see the changes in your browser.
Application entry point - index.js
So we have our application and it's running in our browser. Great! How does all this work and why do we even call a React application a Single Page Application or SPA for short?
A React application's entry point is the index.js file.
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
Okay, that looks like a lot of things going on. Let's take a closer look at the important bits. Line 1 to Line 5 are import statements. We start by importing React
and ReactDOM
which are crucial for the later bits. Then we import index.css as a module (nothing special, just some default styles created by the command line tool), the App
component and a reporting utility which we won't worry about now.
Line 7 to Line 12 is where the magic happens. What React does is it looks for an element with the ID of root and creates the React application root in that element. But where is that element with the root ID? It is located in the only HTML page in the project, which is the index.html file located in the public directory. Here is an extract from that file with the root element.
<body>
<noscript>You need to enable JavaScript to run this app.</noscript>
<div id="root"></div>
<!--
This HTML file is a template.
If you open it directly in the browser, you will see an empty page.
You can add webfonts, meta tags, or analytics to this file.
The build step will place the bundled scripts into the <body> tag.
To begin the development, run `npm start` or `yarn start`.
To create a production bundle, use `npm run build` or `yarn build`.
-->
</body>
On Line 3 we can see a div with the ID of root. This div
will be populated with our entire application!
Ok, back to the index.js file. So Line 7 creates the React root within that element and what lines Line 8 through Line 12 do is render the App
component within this root.
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
What this all ends up doing is basically replacing the contents of that root element (remember the div in the public/index.html file) with the contents of the App
component.
This, right here, is why we call a React application a Single-Page Application (SPA), because there's only one page in the sense of HTML pages and your entire application is rendered within that one tag inside the page. And yes, this even extends to more complicated scenarios like client-side routing (ability to "switch" between pages) where we have the illusion of having multiple pages, but in reality we still do not. It is still the content of the root div element being dynamically changed according to the "page" requested.
The App component
We have briefly mentioned that it is in fact the App
component that gets rendered first. But what is this component? Is it special in any way? Let's take a look.
To answer the previous question right off the bat: no, there is nothing special about the App
component. If we look inside this component we can see that is contains many other HTML elements.
import logo from "./logo.svg";
import "./App.css";
function App() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer">
Learn React
</a>
</header>
</div>
);
}
export default App;
If you were to copy everything between lines Line 6 and Line 20 into the index.js file - in place of the App
component - you would get the same results in your browser.
Then why even bother with using components and what even is a component? We will answer this in the next section.
Introduction to components
Components are the basic building blocks of a React application. A component encapsulates three parts: a HTML layout, styles and some logic. Why use them? Because by extracting certain pieces of our application into components we can reuse them, pass dynamic information into them and in general, make our application more maintainable then in we were to put all the pieces into one file. Let's build a simple greeting component to demonstrate these ideas.
Quick aside: components are named using Pascal Case - meaning each word in the name starts with an uppercase letter. A component uses Pascal Case for both it's filename and it's function name, as we'll see in just a moment.
ExampleThisNameUsesPascalCase because each word starts with an uppercase letter.
Ok, so our component will greet the user by displaying a welcome message and the user's name.
First, create a file called Greeting.js inside the src
directory.

Next, let's create the most basic structure for any component, which is to export a function or a constant with the component's name (in our case this is Greeting
)
import React from "react";
function Greeting() {}
export default Greeting;
We start by importing React from the react module. This is necessary in order to use JSX (JavaScript XML) which is a syntax extension for JavaScript and will be covered in just a moment. All we have so far is an exported function, if you were to ignore the first line this would be nothing more than your average JavaScript file.
Please note: from React version 17 you no longer have to important React in each component (first line). The reason we are showing you it here is because you might work on projects with older React versions.
Another reason is certain tools that generate empty components will also include the React import so it's best to know about it. 🙂
Back to our component, time to add some React-specific stuff! From within a React component you will want to return the HTML structure you would like your component to display. Since we are building a greeting component let's add the welcome message within a paragraph element and make the name bold.
import React from "react";
function Greeting() {
return (
<p>
Welcome, <strong>John</strong>! Good to see you again!
</p>
);
}
export default Greeting;
Ok, what did we do here? We added a return statement for the function followed by a pair of parenthesis and within those parenthesis we have HTML. Normally this would be weird, why would there be HTML inside a JavaScript file? This is JSX - we are extending the JavaScript syntax by allowing HTML to be directly embedded inside it. Pretty cool!
The only problem is, this doesn't show up anywhere. How can we get our component to show on the home page? Well, we have to use it just like any other HTML element - as a tag. We are going to add our newly created Greeting
component within the already existing App
component. To do this, simply open App.js and add Line 3 and Line 9.
import logo from "./logo.svg";
import "./App.css";
import Greeting from "./Greeting";
function App() {
return (
<div className="App">
<header className="App-header">
<Greeting />
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
}
export default App;
In Line 3 we are importing our component. This is nothing special, it's the same kind of import you would do in any JavaScript application. In Line 9 we use our new component just as if it were a normal HTML tag (with the exception of the naming convention). Having done so, go back to your browser and you should see the following results.

Congratulations! You have just created your first React component! 🎉
Remember how we talked about components being re-usable building blocks of our React applications? Well, let's go ahead and re-use it! Add Line 10 and check out the results in the browser.
import logo from "./logo.svg";
import "./App.css";
import Greeting from "./Greeting";
function App() {
return (
<div className="App">
<header className="App-header">
<Greeting />
<Greeting />
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
}
export default App;

Awesome! That's all we needed to do to include another copy of our component. Think about how powerful this can become once you have more complex structures and logic within each component.
There's one problem though ... the name is always the same. Time to introduce props.
Introduction to Props
Props are the primary way of passing data into a component. If you look at our previous example you can see that we handcoded the name "John" (or any other name you picked). This is not exactly what we want. We'd prefer to have a more dynamic functionality capable of display any name. This is where props come in.
In order to pass in props we simply need to pass in attributes. Once again, this is very similar to how we pass in information to HTML tags using like classes and IDs. Let's go ahead and pass in an attribute called name
and give it a value of your choice. I'm going to pass in John
for the first Greeting and Jane
for the second. Feel free to choose any name you like. Here we are passing in string type values. In a bit we will look at passing different types of information like numeric values and objects.
import logo from "./logo.svg";
import "./App.css";
import Greeting from "./Greeting";
function App() {
return (
<div className="App">
<header className="App-header">
<Greeting name="John" />
<Greeting name="Jane" />
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
}
export default App;
What we did here was added an attribute to our Greeting
component. But React won't know what to do with it unless we tell it to. Let's hop back into src/Greeting.js and use the new prop.
import React from "react";
function Greeting(props) {
return (
<p>
Welcome, <strong>{props.name}</strong>! Good to see you again!
</p>
);
}
export default Greeting;
First we add a parameter to our Greeting
component (function) called props
as seen in Line 3. React will take all attributes passed in to the component and make an object out of it and pass that object in the first parameter of the function thus making all attributes available in the props
object.
Then, the way we access a specific prop is by using it's name as a property on the props
object. This can be seen in Line 6. Note the pair the curly braces. These are required in JSX for a value to be interpreted rather than simply printed out. If we were to ommit the curly braces our component would literally show Welcome back, props.name! Good to see you again!
With these modifications done, check back in your browser and see the two lines of greetings are now different with the names you have passed into them.

As we have mentioned earlier, a React component is not much different from your average JavaScript function. As such you can pass in any kind of props into it, even functions! Here are a few examples for different types of data being passed in. Remember, unless you want a literal value to be passed in you will need to use curly braces, this also applies for the passing of the props not just when using them.
<User
email="john@example.com"
personalInformation={{ firstName: "John", lastName: "Doe" }}
age={25}
onEmailUpdate={(newEmail) => console.log(newEmail)}
isVerified={true}
/>
In the example above we use curly braces on all but the first prop (email). This is because, while it is perfect for us that the email will be passed in as type of string, the other attributes have different type. Most notable in the numeric prop age, where the reason for the curly braces is that we don't want the value "25" to be passed as a string, we want it to be of type number.
Styling your components
One approach to styling your components is using *.css
files as modules.
First, create a .css
file with the name of your component. (Naming it the same as your component is only a convention, feel free to use any name you'd like.)ExampleIf your component is called App.jsx, your style file would be App.css.This is an ordinary CSS file, there is nothing special about it so go ahead and fill it in the CSS classes/IDs/any other selectors of your choice.
Next, we need to import this file into our component. Doing so is easy, simply import it as a module.
Exampleimport './App.css'
Having imported the styles, it's time to use them! But first, let's briefly discuss one key difference in using CSS classes here versus in an HTML file. You see, since JSX is essentially JavaScript, we must use the JavaScript API for styling our components (i.e.: using selectors defined in our style file). The way we do this, is by using the className
attribute on our component.
<div className="some-css-class other-css-class and-another-css-class">
Example
</div>
One important thing to note here is that importing the CSS file in our component scopes it to the component. What this means is only that component will have access to the CSS selectors defined in the aforementioned CSS file. This is great as we do not have to worry about having conflicting class names in different components.ExampleIf you have a List
component you could create a CSS class for it's items: .list-item
. You need not worry about whether another component also has a .list-item
style in the stylesheet they import as the two CSS files are scoped to their respected components.
For further ways of styling, please refer to the official documentation above.